This document outlines my contributions to the following software projects:
PROJECT: Anakin
Overview
Anakin is a desktop flashcard management application created by team T09-2 to solve the inherent problems associated with physical flashcards. It aims to facilitate quick and intuitive management of virtual flashcards as well as the sharing of flashcard decks. Additionally, it features the ability to rate the difficulty of individual flashcards and a scheduling algorithm that prompts reviews of cards based on their difficulty rating.
Team T09-2 consisted of myself, Joel Lee, Tay Yu Jia, Foo Guo Wei, and Thanh.
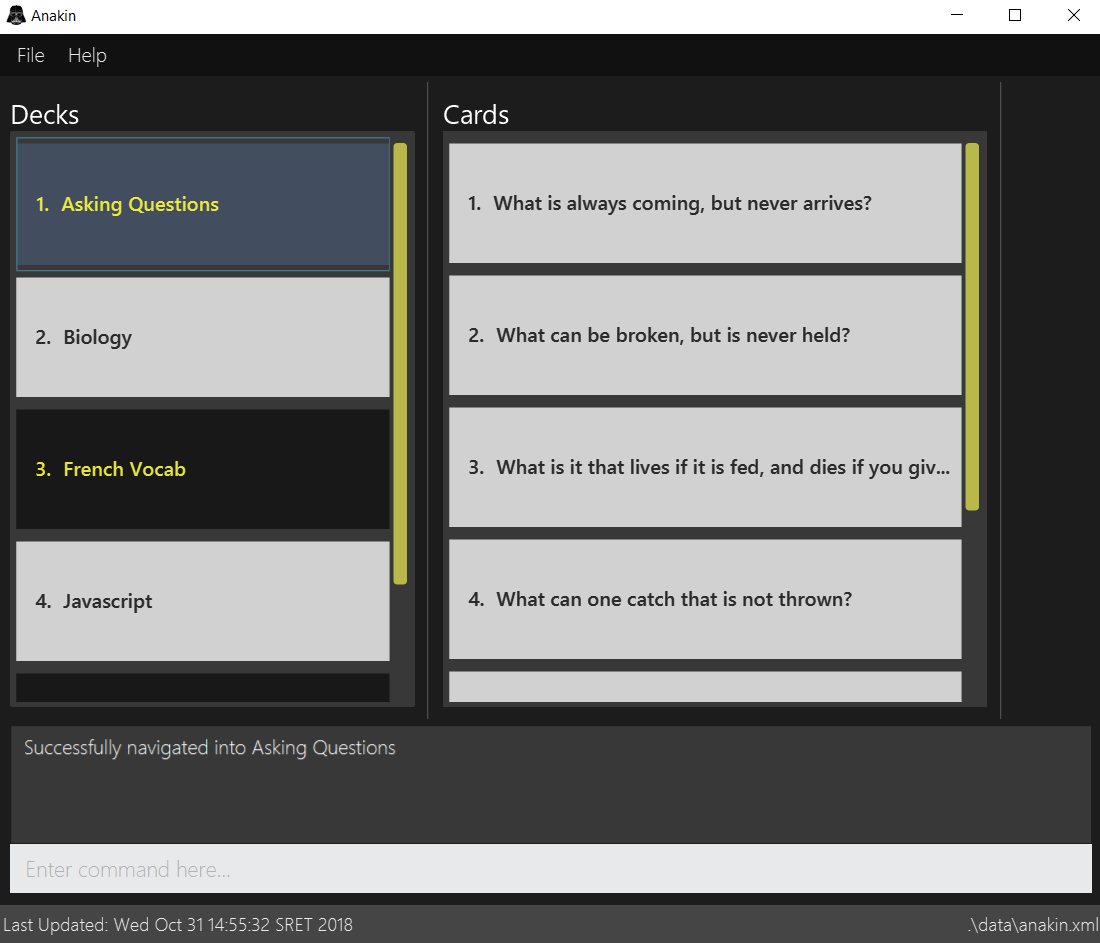
Screenshot of Anakin’s main page
While Anakin features a GUI created with JavaFX, users are only able to interact with Anakin through a commandline interface. Anakin is written in Java, and has about 10 kLoC.
Anakin’s codebase draws from SE-EDU's AddressBook Level 4.
Summary of contributions
-
Area of responsibility: Logic
-
Role: Manage command structure, command execution (by making API calls to the main model), and handling of user input arguments.
-
Integration: Have to work closely with the head of Model, Thanh, to successfully implement most of the features and functionalities in Anakin.
-
-
Major enhancement: added the ability to import/export decks (Pull request: #166)
-
What it does: allows users to export a deck to a specified file location. Also allows the user to import any decks by providing the location of the file.
-
Justification: This feature improves the product significantly because a user can share decks across devices or even with other users.
-
Highlights: This enhancement has a major effect on user experience. It required an in-depth analysis of design alternatives.
-
-
Major enhancement: added the ability to navigate into a deck (Pull request: #62)
-
What it does: allows users to select and enter a deck object.
-
Justification: The UI would be too cluttered if every deck displayed all the cards it contained. Instead we opted to have cards of each hidden until the user enters a deck. Only then will a user be able to see the cards of that deck.
-
Highlights: Provides the necessary framework for separation of deck and card operations. Subsequent card level operations implemented by other team members rely on the framework provided by this feature as card creation, editing and deletion actions are only available if the user has navigated inside a deck.
-
-
Minor enhancement: added a list command for decks that clears the current filters and displays all decks in Anakin.
-
Code contributed: [Functional code tracked by Reposense]
-
Other contributions:
-
Project management:
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Provided below are some of my more notable contributions to the User Guide. |
Export deck to xml file : export
To create an xml
file of the deck at INDEX_OF_DECK.
Format: export INDEX_OF_DECK
Example:
-
1. First, display all the decks in Anakin using
list
.
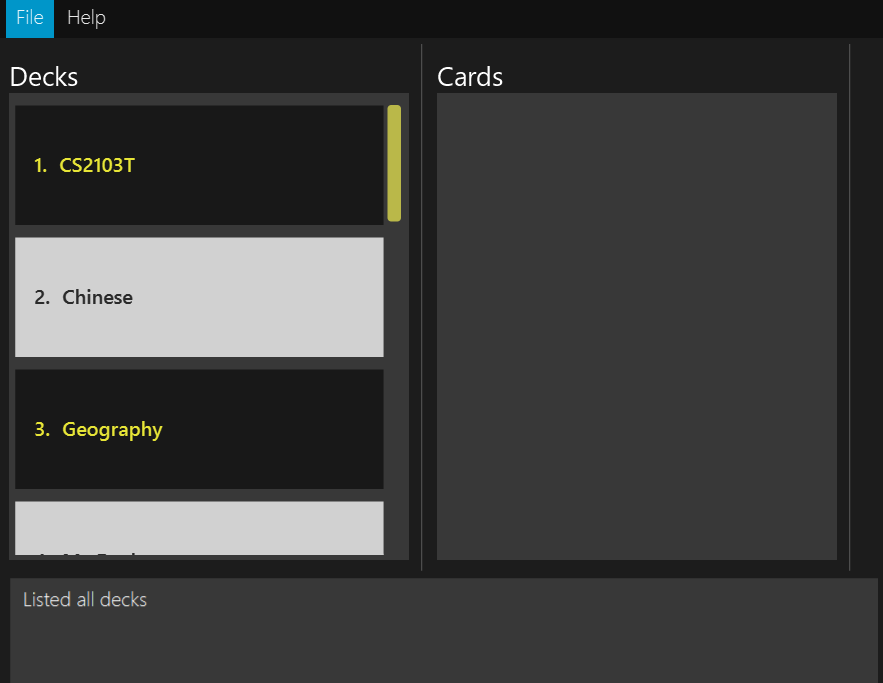
-
2. Say you want to export "Geography" (the 3rd deck), simply enter the command:
export 3
. You should see the following message:

"Geography.xml" will be created in the same directory as the Anakin.jar file.
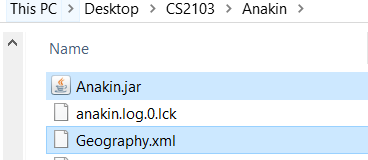
Import deck from xml file : import
To import a deck from the xml
file at the specified FILEPATH.
Format: import FILEPATH
Examples:
Say you want to import a deck called "Geography" and you have the Geography.xml
file in the same folder as Anakin.jar.
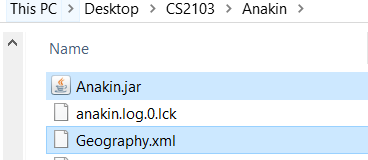
-
Simply enter
import Geography.xml
and Anakin will import the deck "Geography".
Before
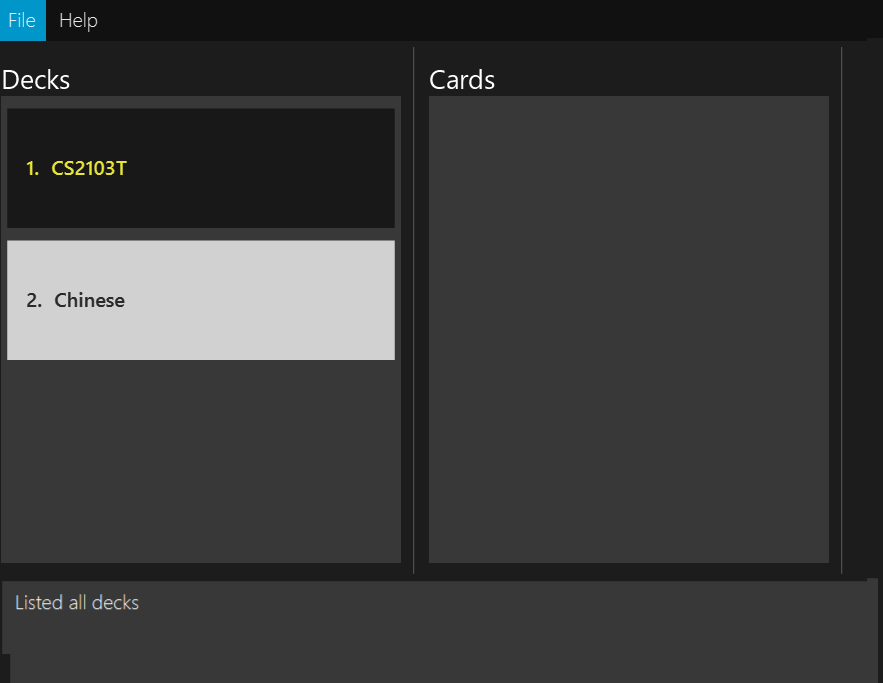
After
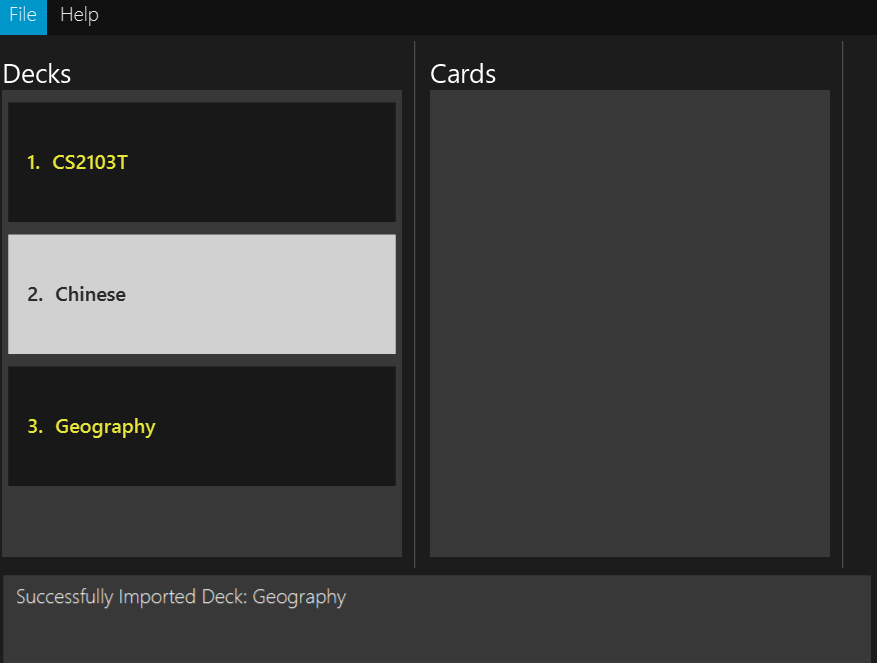
-
If you want to import a deck that is located elsewhere, for example the file is in Desktop, you can enter
import
followed by the full filepath (something like:import C:/Users/Admin/Desktop/My Deck2.xml
) and Anakin will be able to import the deck.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. |
Logic component
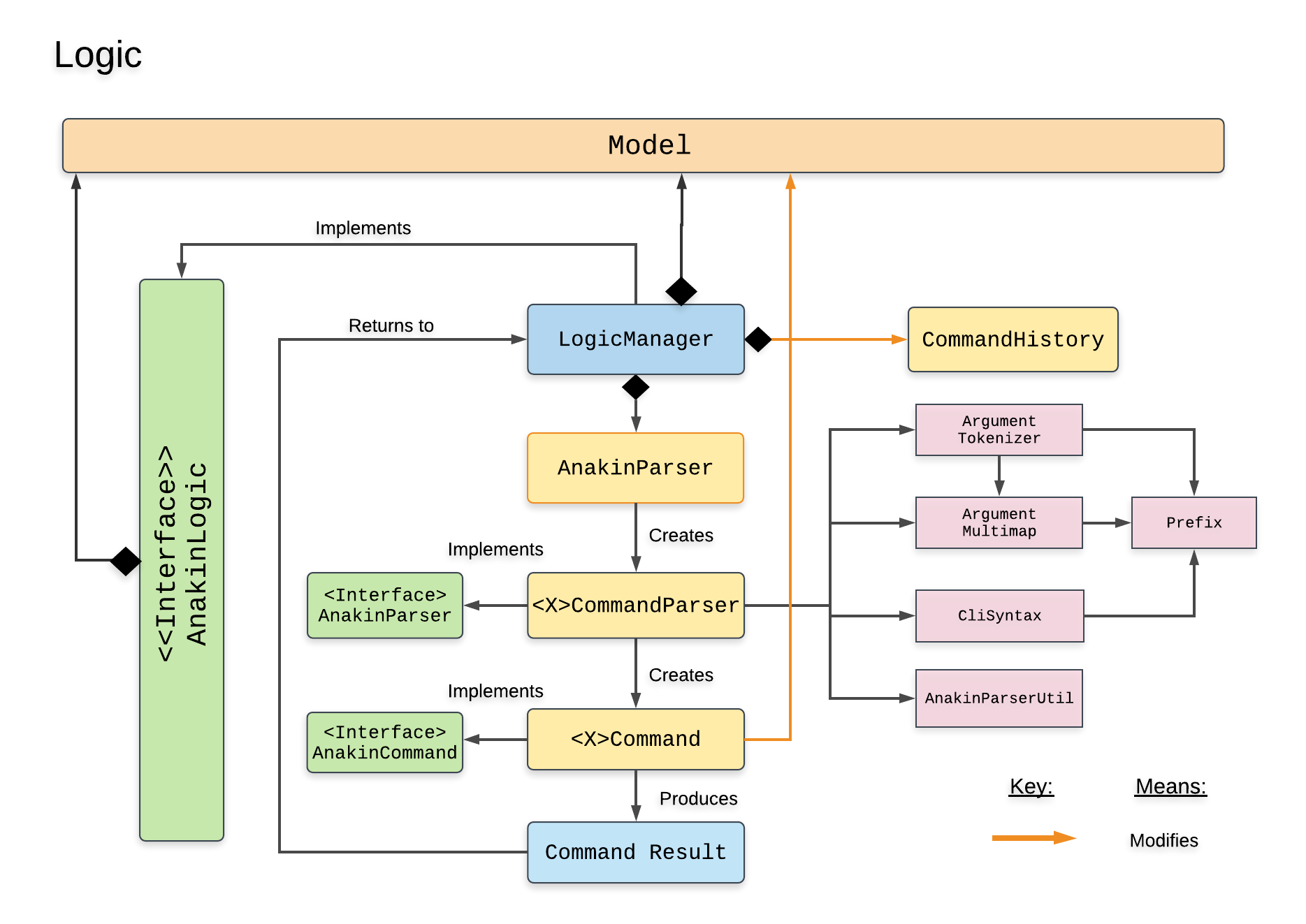
Structure of the Logic component
The 'Logic' component:
The LogicManager
contains an AnakinModel
, an AnakinParser
, and a CommandHistory
.
When a string input by the user is parsed by AnakinParser
, it creates a new AnakinCommand
of the appropriate type with the relevant arguments (based on parser tokens). Each AnakinCommand
has its own Class. LogicManager
will call execute
on the command object. If successful, LogicManager
will modify Model
accordingly. Regardless of success, LogicManager
will update CommandHistory
with the CommandResult
.
Import/Export Implementation
Current implementation (v1.3)
Imports and exports in Anakin are managed by a PortManager.
Exporting a Deck
will create an XmlExportableDeck
, which is exported as a .xml file in the same folder as the Anakin.jar file.
Upon creation, Model will initialize a PortManager. When an ExportCommand or ImportCommand is executed, it will call the Model’s importDeck or exportDeck method.
PortManager uses the java.nio Path
package to navigate directories.
Design considerations
-
Alternative 1 (current choice): Have Model contain a PortManager class to manage imports and export.
-
Explanation: Commands pass their arguments to Model, which passes arguments to the PortManager. In the case of ExportCommand, the
Deck
to be exported is passed from Command to Model to PortManager. PortManager returns aString
of the export location, which is passed to Model, then passed to the Command for printing. -
Pros: Better modularity and Separation of Concerns
-
Cons: Have to pass messages through many layers and methods.
-
-
Alternative 2: Have Model itself manage imports and exports
-
Explanation: Create methods in Model that directly handle conversion, imports, and exports.
-
Pros: Less message passing between layers
-
Cons: Worse modularity and Separation of Concerns.
-
-
Alternative 3: Use a 3rd party library to assist in managing imports/exports
-
Pros: Potentially more powerful functionality.
-
Pros: Good Separation of Concerns as the library is entirely modular.
-
Cons: Need to create methods to adapt data to be compatible with the library API.
-
Cons: Functionality is obscured. May be difficult to fix any unforseen errors.
-
Appendix A: Use Cases
(For all use cases below, the System is Anakin
and the Actor is the user
, unless specified otherwise)
List decks
MSS
-
User requests to list decks
-
Anakin shows a list of decks
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
Create a deck
MSS
-
User requests to create deck
-
Anakin prompts for deck details
-
User enters deck details
-
Anakin creates the deck
Use case ends.
Extensions
-
3a. User enters name of existing deck
-
3a1. Anakin displays an error message.
-
3a2. Anakin prompts for deck details.
Use case resumes at step 4.
-
Delete a deck
MSS
-
User requests to list decks
-
Anakin shows a list of decks
-
User requests to delete a specific deck in the list
-
Anakin deletes the deck
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Anakin displays an error message.
Use case resumes at step 2.
-